Transform portrait aspect to square aspect by adding blurred stripes
Transform portrait aspect to square aspect by adding blurred stripes
Hallo,
I am looking for a simple solution:
The input image has a portrait aspect, i.e. the heigth is larger than the width.
I want to crop stripes from the left and the right side of the input image,
then I want to blur the stripes and montage the stripes left and right to
the input image. The result should be an image of square aspect.
See here for an example:
https://www.instagram.com/p/BK3j9yJBaPl/
DanielDD
I am looking for a simple solution:
The input image has a portrait aspect, i.e. the heigth is larger than the width.
I want to crop stripes from the left and the right side of the input image,
then I want to blur the stripes and montage the stripes left and right to
the input image. The result should be an image of square aspect.
See here for an example:
https://www.instagram.com/p/BK3j9yJBaPl/
DanielDD
-
- Posts: 12159
- Joined: 2010-01-23T23:01:33-07:00
- Authentication code: 1151
- Location: England, UK
Re: Transform portrait aspect to square aspect by adding blurred stripes
What have you tried so far? The operation seems fairly simple:
1. Calculate how much extra width you need on the left and right.
2. Crop that much from the left and right of the input image.
3. Blur these how you want.
4. "+append" these three (the extra left, the input, and the extra right) together.
Step (1) will be slightly different according to your script language. But you haven't bothered to say what language you will use. Nor what version of IM you use.
1. Calculate how much extra width you need on the left and right.
2. Crop that much from the left and right of the input image.
3. Blur these how you want.
4. "+append" these three (the extra left, the input, and the extra right) together.
Step (1) will be slightly different according to your script language. But you haven't bothered to say what language you will use. Nor what version of IM you use.
snibgo's IM pages: im.snibgo.com
Re: Transform portrait aspect to square aspect by adding blurred stripes
ImageMagick 6.9.5-7 Q16 x86_64
I would prefer a tcsh script (cygwin).
I would prefer a tcsh script (cygwin).
-
- Posts: 12159
- Joined: 2010-01-23T23:01:33-07:00
- Authentication code: 1151
- Location: England, UK
Re: Transform portrait aspect to square aspect by adding blurred stripes
I've never used tcsh. Here is a Windows BAT solution, for IM v6:
It doesn't have any error checking (eg if the input isn't portrait, or is too thin).
Code: Select all
set SRC=c.png
for /F "usebackq" %%L in (`%IM%convert ^
-ping %SRC% -format "HH=%%H\nWL=%%[fx:int((h-w)/2)]\nWR=%%[fx:h-w-int((h-w)/2)]" ^
info:`) do set %%L
if "%WL%"=="" exit /B 1
echo HH=%HH% WL=%WL% WR=%WR%
%IM%convert ^
%SRC% ^
( -clone 0 -gravity West -crop %WL%x%HH%+0+0 +repage -blur 0x5 ) ^
( -clone 0 -gravity East -crop %WR%x%HH%+0+0 +repage -blur 0x5 ) ^
-swap 0,1 ^
+append ^
out.png
snibgo's IM pages: im.snibgo.com
- GeeMack
- Posts: 718
- Joined: 2015-12-01T22:09:46-07:00
- Authentication code: 1151
- Location: Central Illinois, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
I'm using ImageMagick 6.9.3 on Windows 10 64. Here's another way to approach this running from a Windows command prompt. This gets pretty close to the example...DanielDD wrote:I want to crop stripes from the left and the right side of the input image, then I want to blur the stripes and montage the stripes left and right to the input image. The result should be an image of square aspect.
Code: Select all
convert input.png ^
( -clone 0 -rotate 90 ) ^
( -clone 1,0 -layers merge ) ^
( -clone 2,0 -gravity east -composite -blur 0x20 ) ^
( -clone 3,0 -gravity center -composite ) ^
-delete 0--2 ^
output.png
- fmw42
- Posts: 25562
- Joined: 2007-07-02T17:14:51-07:00
- Authentication code: 1152
- Location: Sunnyvale, California, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
Here is another way. Unix syntax.
Input:


Or if you want to unfold (mirror) the sides, you can do

You can also use any of the virtual-pixel methods available such as dither.

Or random

Too bad there is not a virtual-pixel option to copy the sides.
Aside: For a more general bordering effect similar to this, see my script imageborder at the link below.
Input:

Code: Select all
convert monet2.jpg \
\( -clone 0 -set option:distort:viewport "%hx%h+0+0" \
-filter point -distort SRT 0 +repage \
-clone 0 -gravity east -compose over -composite \
-blur 0x5 \
-clone 0 -gravity center -compose over -composite \) \
-delete 0 \
monet2_stripes.jpg

Or if you want to unfold (mirror) the sides, you can do
Code: Select all
convert monet2.jpg \
\( -clone 0 -virtual-pixel mirror \
-set option:distort:viewport "%hx%h-%[fx:(h-w)/2]+0" \
-filter point -distort SRT 0 +repage -blur 0x5 \) \
+swap -gravity center -compose over -composite \
monet2_stripes2.jpg

You can also use any of the virtual-pixel methods available such as dither.
Code: Select all
convert monet2.jpg \
\( -clone 0 -virtual-pixel dither \
-set option:distort:viewport "%hx%h-%[fx:(h-w)/2]+0" \
-filter point -distort SRT 0 +repage -blur 0x5 \) \
+swap -gravity center -compose over -composite \
monet2_stripes2.jpg

Or random
Code: Select all
convert monet2.jpg \
\( -clone 0 -virtual-pixel random \
-set option:distort:viewport "%hx%h-%[fx:(h-w)/2]+0" \
-filter point -distort SRT 0 +repage -blur 0x5 \) \
+swap -gravity center -compose over -composite \
monet2_stripes2.jpg

Too bad there is not a virtual-pixel option to copy the sides.
Aside: For a more general bordering effect similar to this, see my script imageborder at the link below.
- GeeMack
- Posts: 718
- Joined: 2015-12-01T22:09:46-07:00
- Authentication code: 1151
- Location: Central Illinois, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
Here's another way to get the same general effect with IM6 from a Windows command prompt...
That just stretches the original image into a square, blurs it, and composites the original over the center. It's actually more like the way it's done in the linked example, but that example background is resized maintaining the aspect ratio, blurred, and cropped to a square.
Edited to add: With ImageMagick 7 the effect in DanielDD's linked example can be virtually duplicated with this command...
Code: Select all
convert input.png ^
( -clone 0 +distort Affine "0,0 0,0 %[w],0 %[h],0 0,%[h] 0,%[h]" -blur 0x20 ) ^
+swap -gravity center -composite output.png
Edited to add: With ImageMagick 7 the effect in DanielDD's linked example can be virtually duplicated with this command...
Code: Select all
magick input.png -write mpr:input -resize %[h]x -blur 0x20 ^
-gravity center -extent %[w]x%[w] mpr:input -composite outputIM7.png
- fmw42
- Posts: 25562
- Joined: 2007-07-02T17:14:51-07:00
- Authentication code: 1152
- Location: Sunnyvale, California, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
FYI:
From what I see in your example, it does not appear to be copying the sides and blurring and adding to make square. It appears to me to have come from a square image that simply has been blurred and the center portion copied over the blurring image. You can see that, since the blurred rails continue along the same slope with no discontinuity in height, which would have occurred if the sides had been copied.
- GeeMack
- Posts: 718
- Joined: 2015-12-01T22:09:46-07:00
- Authentication code: 1151
- Location: Central Illinois, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
I tried several things, mostly to find the simplest, more or less cross platform way to achieve the effect. The example DanielDD linked looks to me like his original image was enlarged to make the height of the original into the width of the resized image, then that enlarged copy was blurred, then the original was composited onto the center, then it was cropped to square. This is the command, with IM7 on Windows 10, that matched the example almost perfectly...fmw42 wrote:From what I see in your example, it does not appear to be copying the sides and blurring and adding to make square. It appears to me to have come from a square image that simply has been blurred and the center portion copied over the blurring image.
Code: Select all
magick input.png -write mpr:input -resize %[h]x -blur 0x20 ^
-gravity center -extent %[w]x%[w] mpr:input -composite outputIM7.png

I'm pretty sure that's how it was done.
- fmw42
- Posts: 25562
- Joined: 2007-07-02T17:14:51-07:00
- Authentication code: 1152
- Location: Sunnyvale, California, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
That could be correct. I had not considered resizing to square first. Can you upload the original image? I do not recall that you provide the input image before, only the example output.
-
- Posts: 12159
- Joined: 2010-01-23T23:01:33-07:00
- Authentication code: 1151
- Location: England, UK
Re: Transform portrait aspect to square aspect by adding blurred stripes
Trim the OP image, extend it to make "holes", and fill them:
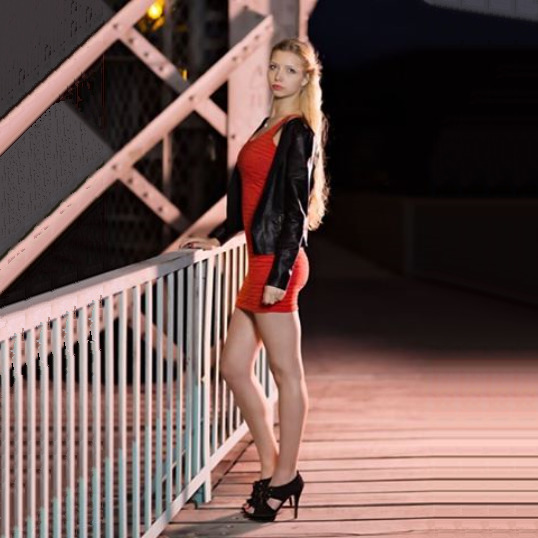
(Definitely not what the OP wanted, but I couldn't resist.)
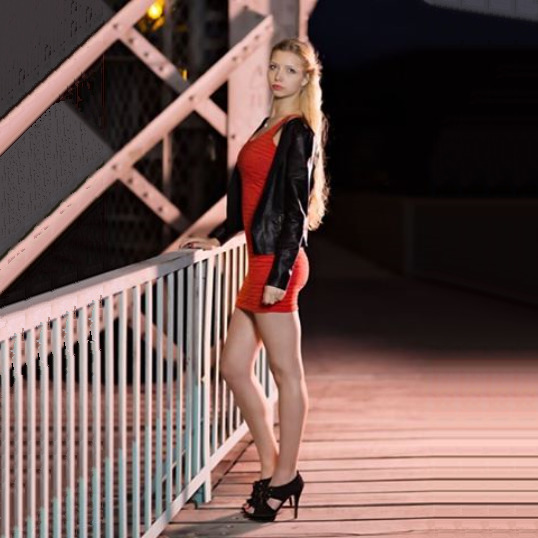
(Definitely not what the OP wanted, but I couldn't resist.)
Code: Select all
%IMDEV%convert ^
bridge.png ^
-crop 332x538+109+0 ^
-background None ^
-gravity Center ^
-extent 538x538 ^
+repage ^
-process 'fillholespri wr 10 lsr 5c als off a v' ^
bridge_fhp.jpg
snibgo's IM pages: im.snibgo.com
- fmw42
- Posts: 25562
- Joined: 2007-07-02T17:14:51-07:00
- Authentication code: 1152
- Location: Sunnyvale, California, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
snibgo:
What was the original input? Is the picture you show your reconstructed original?
What was the original input? Is the picture you show your reconstructed original?
Re: Transform portrait aspect to square aspect by adding blurred stripes
Thank you very much.
I decided to use fmw42's unix-syntax script, because it was easy to include it into an
existing tcsh-script. I already used it in instagram:
https://www.instagram.com/dr.phot.graph/
Daniel
I decided to use fmw42's unix-syntax script, because it was easy to include it into an
existing tcsh-script. I already used it in instagram:
https://www.instagram.com/dr.phot.graph/
Daniel
-
- Posts: 12159
- Joined: 2010-01-23T23:01:33-07:00
- Authentication code: 1151
- Location: England, UK
Re: Transform portrait aspect to square aspect by adding blurred stripes
@Fred: in my fillholespri script, bridge.png is a crop from a screenshot of the link in the OP. I couldn't find a download button. Here is that crop, but as a jpg:
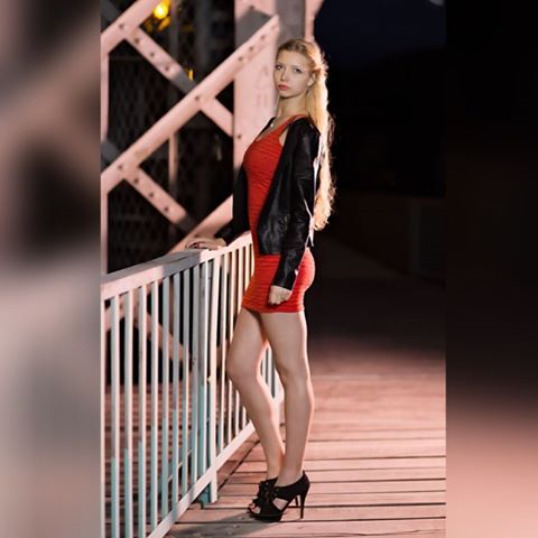
For my fillholespri, I trim off the blurred sides, and a bit more so I don't have a vertical line at the left edge of the picture.
The picture I show is the output from fillholespri.
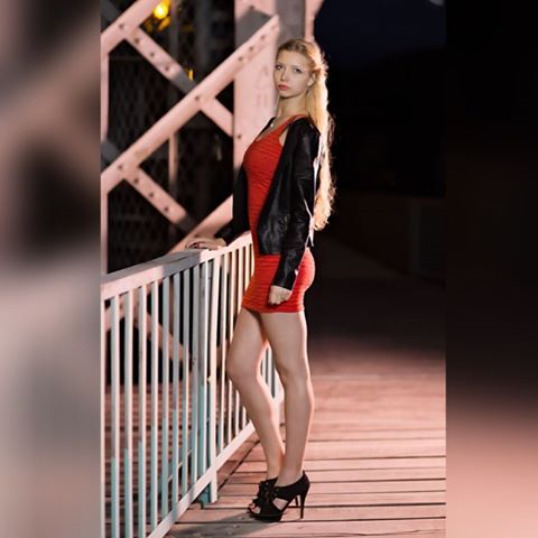
For my fillholespri, I trim off the blurred sides, and a bit more so I don't have a vertical line at the left edge of the picture.
The picture I show is the output from fillholespri.
snibgo's IM pages: im.snibgo.com
- fmw42
- Posts: 25562
- Joined: 2007-07-02T17:14:51-07:00
- Authentication code: 1152
- Location: Sunnyvale, California, USA
Re: Transform portrait aspect to square aspect by adding blurred stripes
Understood. Thanks. Pretty neat recovery of the image. Wish we could get your fillholes (inpainting) code into IM!